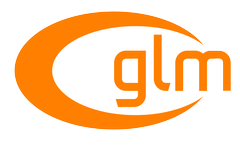 |
1.0.0 API documentation
|
Go to the documentation of this file.
20 #include "../gtc/matrix_transform.hpp"
22 #ifndef GLM_ENABLE_EXPERIMENTAL
23 # error "GLM: GLM_GTX_transform is an experimental extension and may change in the future. Use #define GLM_ENABLE_EXPERIMENTAL before including it, if you really want to use it."
24 #elif GLM_MESSAGES == GLM_ENABLE && !defined(GLM_EXT_INCLUDED)
25 # pragma message("GLM: GLM_GTX_transform extension included")
36 template<
typename T, qualifier Q>
38 vec<3, T, Q>
const& v);
43 template<
typename T, qualifier Q>
44 GLM_FUNC_DECL mat<4, 4, T, Q>
rotate(
46 vec<3, T, Q>
const& v);
51 template<
typename T, qualifier Q>
52 GLM_FUNC_DECL mat<4, 4, T, Q>
scale(
53 vec<3, T, Q>
const& v);
58 #include "transform.inl"
GLM_FUNC_DECL T angle(qua< T, Q > const &x)
Returns the quaternion rotation angle.
GLM_FUNC_DECL mat< 4, 4, T, Q > translate(vec< 3, T, Q > const &v)
Transforms a matrix with a translation 4 * 4 matrix created from 3 scalars.
GLM_FUNC_DECL mat< 4, 4, T, Q > scale(vec< 3, T, Q > const &v)
Transforms a matrix with a scale 4 * 4 matrix created from a vector of 3 components.
GLM_FUNC_DECL mat< 4, 4, T, Q > rotate(T angle, vec< 3, T, Q > const &v)
Builds a rotation 4 * 4 matrix created from an axis of 3 scalars and an angle expressed in radians.