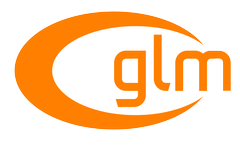 |
1.0.0 API documentation
|
Go to the documentation of this file.
15 #ifndef GLM_ENABLE_EXPERIMENTAL
16 # error "GLM: GLM_GTX_type_trait is an experimental extension and may change in the future. Use #define GLM_ENABLE_EXPERIMENTAL before including it, if you really want to use it."
17 #elif GLM_MESSAGES == GLM_ENABLE && !defined(GLM_EXT_INCLUDED)
18 # pragma message("GLM: GLM_GTX_type_trait extension included")
22 #include "../detail/qualifier.hpp"
23 #include "../gtc/quaternion.hpp"
24 #include "../gtx/dual_quaternion.hpp"
34 static bool const is_vec =
false;
35 static bool const is_mat =
false;
36 static bool const is_quat =
false;
37 static length_t
const components = 0;
38 static length_t
const cols = 0;
39 static length_t
const rows = 0;
42 template<length_t L,
typename T, qualifier Q>
43 struct type<vec<L, T, Q> >
45 static bool const is_vec =
true;
46 static bool const is_mat =
false;
47 static bool const is_quat =
false;
48 static length_t
const components = L;
51 template<length_t C, length_t R,
typename T, qualifier Q>
52 struct type<mat<C, R, T, Q> >
54 static bool const is_vec =
false;
55 static bool const is_mat =
true;
56 static bool const is_quat =
false;
57 static length_t
const components = C;
58 static length_t
const cols = C;
59 static length_t
const rows = R;
62 template<
typename T, qualifier Q>
63 struct type<qua<T, Q> >
65 static bool const is_vec =
false;
66 static bool const is_mat =
false;
67 static bool const is_quat =
true;
68 static length_t
const components = 4;
71 template<
typename T, qualifier Q>
72 struct type<tdualquat<T, Q> >
74 static bool const is_vec =
false;
75 static bool const is_mat =
false;
76 static bool const is_quat =
true;
77 static length_t
const components = 8;
83 #include "type_trait.inl"