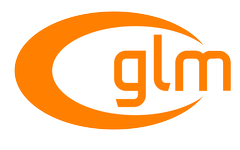 |
0.9.9 API documenation
|
Go to the documentation of this file.
15 #ifndef GLM_ENABLE_EXPERIMENTAL
16 # error "GLM: GLM_GTX_type_trait is an experimetal extension and may change in the future. Use #define GLM_ENABLE_EXPERIMENTAL before including it, if you really want to use it."
20 #include "../detail/type_vec2.hpp"
21 #include "../detail/type_vec3.hpp"
22 #include "../detail/type_vec4.hpp"
23 #include "../detail/type_mat2x2.hpp"
24 #include "../detail/type_mat2x3.hpp"
25 #include "../detail/type_mat2x4.hpp"
26 #include "../detail/type_mat3x2.hpp"
27 #include "../detail/type_mat3x3.hpp"
28 #include "../detail/type_mat3x4.hpp"
29 #include "../detail/type_mat4x2.hpp"
30 #include "../detail/type_mat4x3.hpp"
31 #include "../detail/type_mat4x4.hpp"
32 #include "../gtc/quaternion.hpp"
33 #include "../gtx/dual_quaternion.hpp"
35 #if GLM_MESSAGES == GLM_MESSAGES_ENABLED && !defined(GLM_EXT_INCLUDED)
36 # pragma message("GLM: GLM_GTX_type_trait extension included")
44 template <
template <
typename, precision>
class genType,
typename T, precision P>
47 static bool const is_vec =
false;
48 static bool const is_mat =
false;
49 static bool const is_quat =
false;
50 static length_t
const components = 0;
51 static length_t
const cols = 0;
52 static length_t
const rows = 0;
55 template <
typename T, precision P>
56 struct type<tvec1, T, P>
58 static bool const is_vec =
true;
59 static bool const is_mat =
false;
60 static bool const is_quat =
false;
67 template <
typename T, precision P>
68 struct type<tvec2, T, P>
70 static bool const is_vec =
true;
71 static bool const is_mat =
false;
72 static bool const is_quat =
false;
79 template <
typename T, precision P>
80 struct type<tvec3, T, P>
82 static bool const is_vec =
true;
83 static bool const is_mat =
false;
84 static bool const is_quat =
false;
91 template <
typename T, precision P>
92 struct type<tvec4, T, P>
94 static bool const is_vec =
true;
95 static bool const is_mat =
false;
96 static bool const is_quat =
false;
103 template <
typename T, precision P>
104 struct type<tmat2x2, T, P>
106 static bool const is_vec =
false;
107 static bool const is_mat =
true;
108 static bool const is_quat =
false;
117 template <
typename T, precision P>
118 struct type<tmat2x3, T, P>
120 static bool const is_vec =
false;
121 static bool const is_mat =
true;
122 static bool const is_quat =
false;
131 template <
typename T, precision P>
132 struct type<tmat2x4, T, P>
134 static bool const is_vec =
false;
135 static bool const is_mat =
true;
136 static bool const is_quat =
false;
145 template <
typename T, precision P>
146 struct type<tmat3x2, T, P>
148 static bool const is_vec =
false;
149 static bool const is_mat =
true;
150 static bool const is_quat =
false;
159 template <
typename T, precision P>
160 struct type<tmat3x3, T, P>
162 static bool const is_vec =
false;
163 static bool const is_mat =
true;
164 static bool const is_quat =
false;
173 template <
typename T, precision P>
174 struct type<tmat3x4, T, P>
176 static bool const is_vec =
false;
177 static bool const is_mat =
true;
178 static bool const is_quat =
false;
187 template <
typename T, precision P>
188 struct type<tmat4x2, T, P>
190 static bool const is_vec =
false;
191 static bool const is_mat =
true;
192 static bool const is_quat =
false;
201 template <
typename T, precision P>
202 struct type<tmat4x3, T, P>
204 static bool const is_vec =
false;
205 static bool const is_mat =
true;
206 static bool const is_quat =
false;
215 template <
typename T, precision P>
216 struct type<tmat4x4, T, P>
218 static bool const is_vec =
false;
219 static bool const is_mat =
true;
220 static bool const is_quat =
false;
229 template <
typename T, precision P>
230 struct type<tquat, T, P>
232 static bool const is_vec =
false;
233 static bool const is_mat =
false;
234 static bool const is_quat =
true;
241 template <
typename T, precision P>
242 struct type<tdualquat, T, P>
244 static bool const is_vec =
false;
245 static bool const is_mat =
false;
246 static bool const is_quat =
true;
256 #include "type_trait.inl"