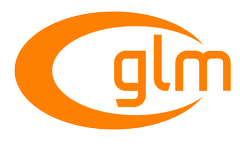 |
1.0.0 API documentation
|
Go to the documentation of this file.
16 #include "../gtc/bitfield.hpp"
18 #ifndef GLM_ENABLE_EXPERIMENTAL
19 # error "GLM: GLM_GTX_bit is an experimental extension and may change in the future. Use #define GLM_ENABLE_EXPERIMENTAL before including it, if you really want to use it."
20 #elif GLM_MESSAGES == GLM_ENABLE && !defined(GLM_EXT_INCLUDED)
21 # pragma message("GLM: GLM_GTX_bit extension included")
30 template<
typename genIUType>
34 template<
typename genIUType>
40 template<length_t L,
typename T, qualifier Q>
48 template<
typename genIUType>
56 template<length_t L,
typename T, qualifier Q>
57 GLM_DEPRECATED GLM_FUNC_DECL vec<L, T, Q>
powerOfTwoAbove(vec<L, T, Q>
const& value);
64 template<
typename genIUType>
72 template<length_t L,
typename T, qualifier Q>
73 GLM_DEPRECATED GLM_FUNC_DECL vec<L, T, Q>
powerOfTwoBelow(vec<L, T, Q>
const& value);
80 template<
typename genIUType>
88 template<length_t L,
typename T, qualifier Q>
GLM_DEPRECATED GLM_FUNC_DECL vec< L, T, Q > powerOfTwoNearest(vec< L, T, Q > const &value)
Return the power of two number which value is the closet to the input value.
GLM_FUNC_DECL vec< L, T, Q > highestBitValue(vec< L, T, Q > const &value)
Find the highest bit set to 1 in a integer variable and return its value.
GLM_FUNC_DECL genIUType lowestBitValue(genIUType Value)
GLM_DEPRECATED GLM_FUNC_DECL vec< L, T, Q > powerOfTwoAbove(vec< L, T, Q > const &value)
Return the power of two number which value is just higher the input value.
GLM_DEPRECATED GLM_FUNC_DECL vec< L, T, Q > powerOfTwoBelow(vec< L, T, Q > const &value)
Return the power of two number which value is just lower the input value.