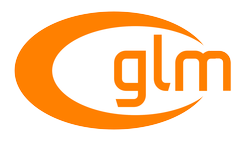 |
1.0.0 API documentation
|
Go to the documentation of this file.
23 #include "../gtc/constants.hpp"
24 #include "../geometric.hpp"
25 #include "../trigonometric.hpp"
26 #include "../matrix.hpp"
28 #if GLM_MESSAGES == GLM_ENABLE && !defined(GLM_EXT_INCLUDED)
29 # pragma message("GLM: GLM_EXT_matrix_integer extension included")
47 template<length_t C, length_t R,
typename T, qualifier Q>
48 GLM_FUNC_DECL mat<C, R, T, Q>
matrixCompMult(mat<C, R, T, Q>
const& x, mat<C, R, T, Q>
const& y);
61 template<length_t C, length_t R,
typename T, qualifier Q>
62 GLM_FUNC_DECL
typename detail::outerProduct_trait<C, R, T, Q>::type
outerProduct(vec<C, T, Q>
const& c, vec<R, T, Q>
const& r);
73 template<length_t C, length_t R,
typename T, qualifier Q>
74 GLM_FUNC_DECL
typename mat<C, R, T, Q>::transpose_type
transpose(mat<C, R, T, Q>
const& x);
85 template<length_t C, length_t R,
typename T, qualifier Q>
91 #include "matrix_integer.inl"
GLM_FUNC_DECL mat< C, R, T, Q > matrixCompMult(mat< C, R, T, Q > const &x, mat< C, R, T, Q > const &y)
Multiply matrix x by matrix y component-wise, i.e., result[i][j] is the scalar product of x[i][j] and...
GLM_FUNC_DECL mat< C, R, T, Q >::transpose_type transpose(mat< C, R, T, Q > const &x)
Returns the transposed matrix of x.
GLM_FUNC_DECL T determinant(mat< C, R, T, Q > const &m)
Return the determinant of a squared matrix.
GLM_FUNC_DECL detail::outerProduct_trait< C, R, T, Q >::type outerProduct(vec< C, T, Q > const &c, vec< R, T, Q > const &r)
Treats the first parameter c as a column vector and the second parameter r as a row vector and does a...