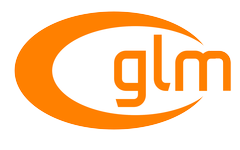 |
1.0.0 API documentation
|
Go to the documentation of this file.
17 #include "../common.hpp"
19 #if GLM_MESSAGES == GLM_ENABLE && !defined(GLM_EXT_INCLUDED)
20 # pragma message("GLM: GLM_EXT_scalar_common extension included")
34 GLM_FUNC_DECL T
min(T a, T b, T c);
42 GLM_FUNC_DECL T
min(T a, T b, T c, T d);
50 GLM_FUNC_DECL T
max(T a, T b, T c);
58 GLM_FUNC_DECL T
max(T a, T b, T c, T d);
67 GLM_FUNC_DECL T
fmin(T a, T b);
76 GLM_FUNC_DECL T
fmin(T a, T b, T c);
85 GLM_FUNC_DECL T
fmin(T a, T b, T c, T d);
94 GLM_FUNC_DECL T
fmax(T a, T b);
103 GLM_FUNC_DECL T
fmax(T a, T b, T C);
112 GLM_FUNC_DECL T
fmax(T a, T b, T C, T D);
119 template<
typename genType>
120 GLM_FUNC_DECL genType
fclamp(genType x, genType minVal, genType maxVal);
127 template<
typename genType>
128 GLM_FUNC_DECL genType
clamp(genType
const& Texcoord);
135 template<
typename genType>
136 GLM_FUNC_DECL genType
repeat(genType
const& Texcoord);
143 template<
typename genType>
151 template<
typename genType>
163 template<
typename genType>
164 GLM_FUNC_DECL
int iround(genType
const& x);
175 template<
typename genType>
176 GLM_FUNC_DECL uint
uround(genType
const& x);
181 #include "scalar_common.inl"
GLM_FUNC_DECL genType mirrorClamp(genType const &Texcoord)
Simulate GL_MIRRORED_REPEAT OpenGL wrap mode.
GLM_FUNC_DECL T min(T a, T b, T c, T d)
Returns the minimum component-wise values of 4 inputs.
GLM_FUNC_DECL T fmin(T a, T b, T c, T d)
Returns the minimum component-wise values of 4 inputs.
GLM_FUNC_DECL genType repeat(genType const &Texcoord)
Simulate GL_REPEAT OpenGL wrap mode.
GLM_FUNC_DECL genType fclamp(genType x, genType minVal, genType maxVal)
Returns min(max(x, minVal), maxVal) for each component in x.
GLM_FUNC_DECL uint uround(genType const &x)
Returns a value equal to the nearest integer to x.
GLM_FUNC_DECL genType mirrorRepeat(genType const &Texcoord)
Simulate GL_MIRROR_REPEAT OpenGL wrap mode.
GLM_FUNC_DECL int iround(genType const &x)
Returns a value equal to the nearest integer to x.
GLM_FUNC_DECL T fmax(T a, T b, T C, T D)
Returns the maximum component-wise values of 4 inputs.
GLM_FUNC_DECL T max(T a, T b, T c, T d)
Returns the maximum component-wise values of 4 inputs.
GLM_FUNC_DECL genType clamp(genType const &Texcoord)
Simulate GL_CLAMP OpenGL wrap mode.